The Bay of Bengal is hit frequently by cyclones. The months of November and May, in particular, are dangerous in this regard.
728x90
반응형
1. if문
조건문은 프로그래밍에서 가장 기본적인 구문 중 하나입니다.
조건문을 사용하면 프로그램이 특정 조건에 따라서 다른 동작을 하도록 할 수 있습니다.
{
// false : 0, null, undefined, false ""(빈문자열)
// true : 1, 2, "0", "1", "ABC", [], {}, true
if(조건식){
document.write("실행되었습니다.(true)");
} else {
document.write("실행되었습니다.(false)");
}
}
결과값 :
실행되었습니다.(true)
실행되었습니다.(false)
2. if문 생략
코드를 더 간결하고 읽기 쉽게 만들기 위해 괄호를 생략할 수 있다.
{
const num = 100;
// if(num){
// document.write("실행되었습니다.(true)");
// } else {
// document.write("실행되었습니다.(false)");
// }
if(num) document.write("실행되었습니다.(true)");
else document.write("실행되었습니다.(false)");
}
결과값 : 실행되었습니다.(true)
3. 삼항 연산자
if문을 사용하지 않고 연산자만으로 조건을 체크할 수 있습니다.
{
const num = 100;
// if(num == 100){
// document.write("true");
// } else {
// document.write("false");
// }
(num == 100) ? document.write("true") : document.write("false");
}
결과값 : true
4. 다중 if(else if)
else if문 안에 또 다른 if문이나 else if문을 사용할 수 있습니다.
{
const num = 100;
if(num == 90){
document.write("실행되었습니다(num == 90)");
} else if(num == 100){
document.write("실행되었습니다(num == 100)");
} else if(num == 110){
document.write("실행되었습니다(num == 110)");
} else if(num == 120){
document.write("실행되었습니다(num == 120)");
} else {
document.write("실행되었습니다");
}
}
결과값 : 실행되었습니다(num == 100)
5. 중첩 if
if문 안에 if문이 포함된 구조입니다.
{
const num = 100;
if(num == 100){
document.write("실행되었습니다.(1)");
if(num == 100){
document.write("실행되었습니다.(2)");
if(num == 100){
document.write("실행되었습니다.(3)");
}
}
} else {
document.write("실행되었습니다.(4)")
}
}
결과값 :
실행되었습니다(1)
실행되었습니다(2)
실행되었습니다(3)
6. switch문
switch의 매개변수 값과 일치하는 case 변수를 찾아 해당 블록의 소스를 실행합니다.
{
const num = 100;
switch(num){
case 90:
document.write("실행90");
break;
case 80:
document.write("실행80");
break;
case 70:
document.write("실행70");
break;
case 60:
document.write("실행60");
break;
case 50:
document.write("실행50");
break;
default:
document.write("0");
}
결과값 : 0
7. while문
조건이 참일 경우 반복적으로 블록을 실행합니다.
{
for(let i=0; i<5; i++){
document.write(i);
}
let num = 0;
while(num<5){
document.write(num);
num++;
}
}
결과값 :
01234
01234
8. do while문
while문과 유사한 반복문입니다. 코드블록을 먼저 실행한 후 조건을 체크합니다.
{
let num2 = 0;
do {
document.write(num2);
num2++;
} while (num2<5);
}
결과값 : 01234
9. for문
for문을 사용해서 1부터 25까지 출력하기
{
let num = 0;
for(let i=1; i<=25; i++){
document.write(i);
num++;
}
document.write(num);
}
결과값 : 12345678910111213141516171819202122232425
10. 중첩 for문
중첩 for문을 사용해서 테이블 만들기
{
let table = "<table border='1'>";
for(let i=0; i<;5; i++){
table += "<tr>";
for(let j=0; j<5; j++){
table += "<td>"+j+"</td>";
}
table += "</tr>";
}
table += "</table>";
document.write(table);
}
결과값 :
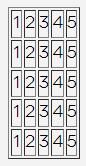
11. break문
조건을 만족할 때 반복문을 강제로 종료합니다.
{
for(let i=1; i<20; i++){
if(i == 10){
break;
}
document.write(i);
}
for(let i=1; i<20; i++){
document.write(i);
if(i == 10){
break;
}
}
}
결과값 :
123456789
12345678910
12. continue문
반복문을 실행하는 중 continue를 만나면 건너뛰고 다음차례의 for문을 실행합니다.
{
for(let i=1; i<;20; i++){
if(i == 10){
continue;
}
document.write(i);
}
for(let i=1; i<20; i++){
document.write(i);
if(i == 10){
continue;
}
}
}
결과값 :
123456789111213141516171819
1234567891011213141516171819